The introduction of React Hooks at React Conf October 2018 was received with great enthusiasm by the React Community. “Why?” you might ask.
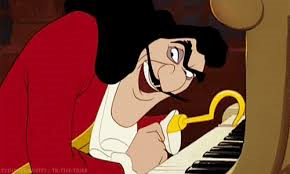
Hooks allow us to write function components that make use of all the functionality and features that used to be only accessible with class components. Function components were once called functional stateless components, but now the use of state is available to these components with React Hooks.
The main motivation behind the invention of React Hooks by the Core React Team was to introduce state management and side-effects in function components without needing to refactor them into class components as they did before.
Side-effects and Lifecycle Methods
In React class components, side-effects were implemented in lifecycle methods (e.g. componentDidMount, componentDidUpdate, componentWillUnmount). What happened if you needed to use more than one side-effect? They would be grouped by the respective lifecycle method.
This changes with Hooks: you can encase a side-effect in one hook with the respective setup and clean up steps.
But fear not: Hooks are backward-compatible!
Let’s see how Hooks work with two examples: the State Hook and the Effect Hook.
Using the State Hook
One of the most used hooks is: useState()
An example of a class component that has state management:
But what if we applied the useState Hook?
We applied the hook with:
What does useState() offer us?
- A variable that holds the state value: count
- A function to change the value: setCount
What about this line of code?
The state hook variable count was placed within { } and, after it’s run as Javascript, the count is rendered.
Let’s compare it with the way it was done in the class-component example shown before with a state variable:
So with useState, we don’t need to use this. Much better right?
What about the following line?
In this line, the setCount function is used to change the count variable.
If the button is clicked then the count variable is increased by 1. As this constitutes a state change, a rendering is then triggered and an update of the view and the new count value.
But what if we need to use multiple state variables?
Here is a small example:
We are using 3 different independent state objects. If we need to update the number, we’ll call the setNumber() function. The same goes for count and title.
Using the Effect Hook
The state Hook allows us to manage the state. But what about side-effects?
An example of a side-effect can be an interaction with the Browser/DOM API or an external API to fetch data.
As discussed earlier, before when we were building functional components we couldn’t access the lifecycle methods in React. This can be changed by applying the Effect Hook.
Once again let’s compare a class component:
And now apply the Effect Hook:
How does the effect hook work? Well, by default the effect hook runs on every render and re-render. If a change of state or in props triggers a new render, how can we guarantee it only runs when the component mounts?
We can make use of the second parameter in useEffect(): an array that lists the things that will cause the hook to run:
- If the array is empty the hook runs once on mount
- If the array contains a prop, for example, a change on this prop will trigger the hook to run
What about the equivalent to componentWillUnmount()? We can just return a function from the effect hook:
Mixing
You can use the State and Effect Hooks together and reap both the benefits.
For example:
Other Hooks
There are also other hooks that derive from the ones mentioned before or that are meant to be used in very specific cases. For example: use Reducer, useCallBack, and useMemo.
You can learn more about these in the respective section in the React official docs.
Custom Hooks
You can also build a custom hook that extracts component logic into reusable functions.
It’s a Javascript function that starts with use and that can also call other hooks and make use of them.
You can also learn more about them including checking an example here.
Summary
My hope is that after reading this article you can start incorporating Hooks in your React code and take advantage of their enormous potential. React Hooks are reusable and there is an extensive number of custom hooks made available by other developers that you can employ.
Links you can check to learn more about React Hooks:
React Tutorial: Components, Hooks and Performance